The previous few years turned out to be surprisingly busy for me. Thus, I didn't write much in the blog.
Now, as things are slowing down, I will try to post more.
In this post, I'd like to mention a small trick for Salesforce development that I hope you will find helpful, especially if you're new to the SFDC platform.
The trick concerns rendering standard Salesforce dashboards inside the Lightning Web Components.
Out of the box, Salesforce has only the Aura component to display the dashboard. Unfortunately, no component is available in the Lightning Web Component library.
Turns out, it is pretty easy to embed the dashboard via the iframe.
All you need to do is to obtain the dashboard ID you want to render, generate the dashboard URL, and add the iframe to the LWC HTML markup.
In the component class, construct the dashboard URL as follows.
import { LightningElement, api, wire, track } from 'lwc';
import userId from '@salesforce/user/Id';
export default class DashboardComponent {
@api dashboardId;
get dashboardUrl() {
return `/desktopDashboards/dashboardApp.app?dashboardId=${this.dashboardId}&displayMode=view&networkId=000000000000000&userId=${userId}`
}
}
You would need to have the currently logged-in user ID. You can easily import it from the User scoped module.
You can expose the dashboard ID property to the builder using the metadata.
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__AppPage</target>
<target>lightning__HomePage</target>
<target>lightning__RecordPage</target>
<target>lightningCommunity__Page</target>
<target>lightningCommunity__Default</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__AppPage">
<property name="dashboardId" label="Dashboard Id" type="String" />
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
Then you need to add the following markup to the HTML template.
<iframe title="dashboard"
height="400px"
width="100%"
frameborder="0"
src={dashboardUrl}
spellcheck="false"
></iframe>
You need to specify the dimensions of the embedded iframe on the page. Here I use 100% width and 400px height.
Unfortunately, we don't know the actual size of the content within the dashboard, so height is static, and it will have the inner scroll in it by default.
Alternatively, you can make it fill the full height of the parent container or use the CSS calc(100vh - {offsetTop})
function to fill the rest of the space on the screen.
There are some libraries to calculate the content inside embedded iframes. You can also achieve that using JavaScript by dynamically setting the iframe's height on load via contentWindow.document.body.scrollHeight
.
For instance, in the LWC, you can get the contentWindow
and inner elements through the refs. You can read more about it here.
You can add the lwc:ref="iframe"
attribute to the iframe and then access its content in the controller via this.refs.iframe
.
But unfortunately, I couldn't find a way to detect when the dashboard content finishes loading to set the height correctly.
So, as a final note, I'd suggest using a more static approach until there is a way to ensure we know the inner content height without polling, etc., that can affect the performance.
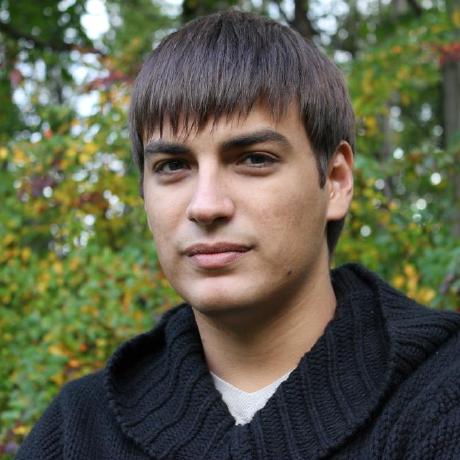
Nikita Verkhoshintcev
Salesforce Consultant
Senior Salesforce and full-stack web developer. I design and build modern Salesforce, React, and Angular applications for enterprises. Usually, companies hire me when complex implementation, custom development and UI is required.